What is the Template Method Design Pattern?
In simple words, a template method design pattern is a behavioral design pattern that defines the basic skeleton (steps) of an algorithm. In this pattern, we can change the behavior of individual steps in the derived class but can not change the sequence by which each method will be called. We can understand it better by looking at the following example.
Real-world example
Let’s suppose that we have to make a burger and it requires some pre-defined steps. So the algorithm (steps) to make a burger is fixed but we can customize each step based on the burger we want to make. Basically, we have a standard procedure (a template) on how to make a burger.
Structural Code
We have a base class named MakeBurger.cs which contains all the basic steps that we need to perform to make a burger. It can have both abstract (when we don’t have default implementation) or virtual (when we have default implementation) methods based on the requirement.
public abstract class MakeBurger
{
public void Prepare()
{
SelectBun();
AddPatty();
AddSauces();
Pack();
}
public abstract void SelectBun();
public abstract void AddPatty();
public abstract void AddSauces();
public virtual void Pack() {
// pack the burger
}
}
Now we will inherit this class in our subclass and override what each step would do.
public class MakeChickenBurger : MakeBurger {
public override void SelectBun() {
// select bun
}
public override void AddPatty() {
// add chicken patty
}
public override void AddSauces() {
// add sauces
}
}
public class MakePotatoBurger : MakeBurger {
public override void SelectBun() {
// select bun
}
public override void AddPatty() {
// add potato patty
}
public override void AddSauces() {
// add sauces
}
}
Now to finally make our burgers we would do.
public MakeBurger Burger(bool isVegBurger) {
if (isVegBurger)
{
MakePotatoBurger burger = new();
burger.Prepare();
return burger;
}
else
{
MakeChickenBurger burger = new();
burger.Prepare();
return burger;
}
}
So here we have it, a simple and basic implementation of a template method design pattern where we define a template inside a base class and let subclasses decide what to do with the methods defined in the class.
Any other way we could put the Template method design pattern to use? Let me know in the comments below. Till then, Happy Coding! 👨💻
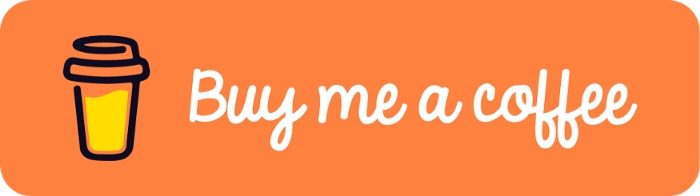
Pingback: Fluent Builder Design Pattern in C#