I was reading a .NET documentation about some common C# code conventions we should know about. In this article, I will summarise and share some key conventions you should follow.
Use string interpolation to concatenate short strings, as shown in the following code.
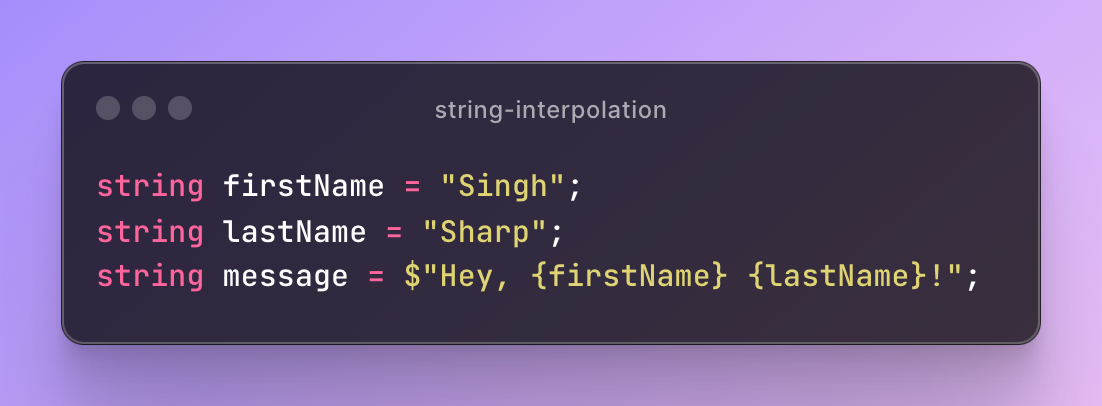
Use one of the concise forms of object instantiation, as shown in the following declarations.
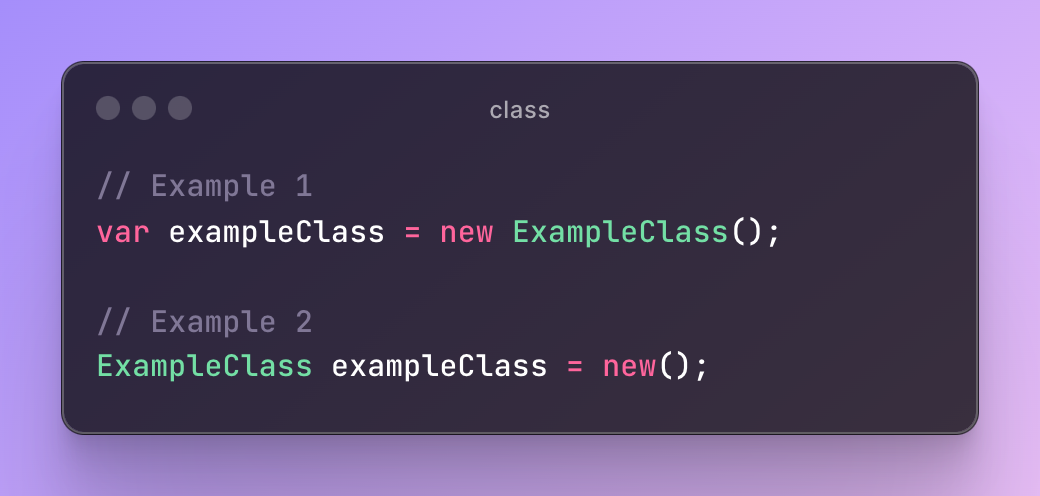
Use where
clauses before other query clauses to ensure that later query clauses operate on the reduced, filtered set of data.
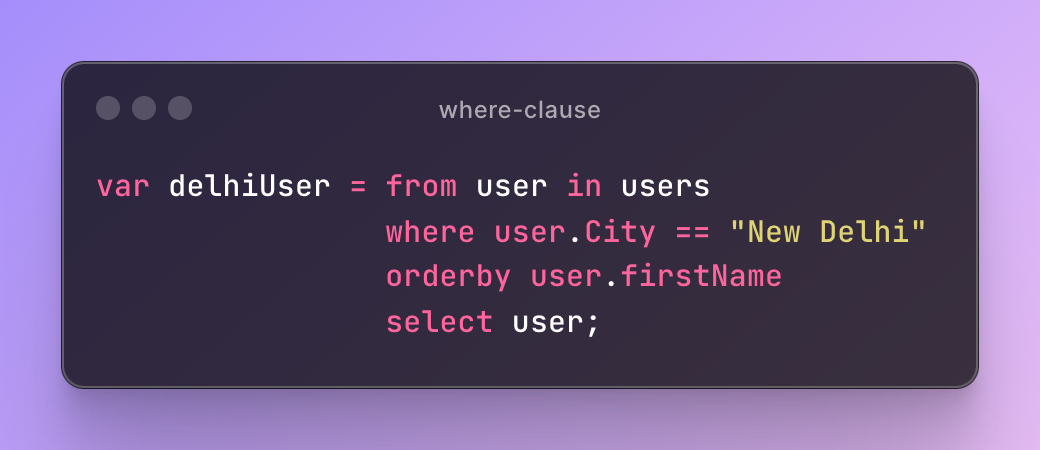
To append strings in loops, especially when you are working with large amounts of text, use a System.Text.StringBuilder
object.
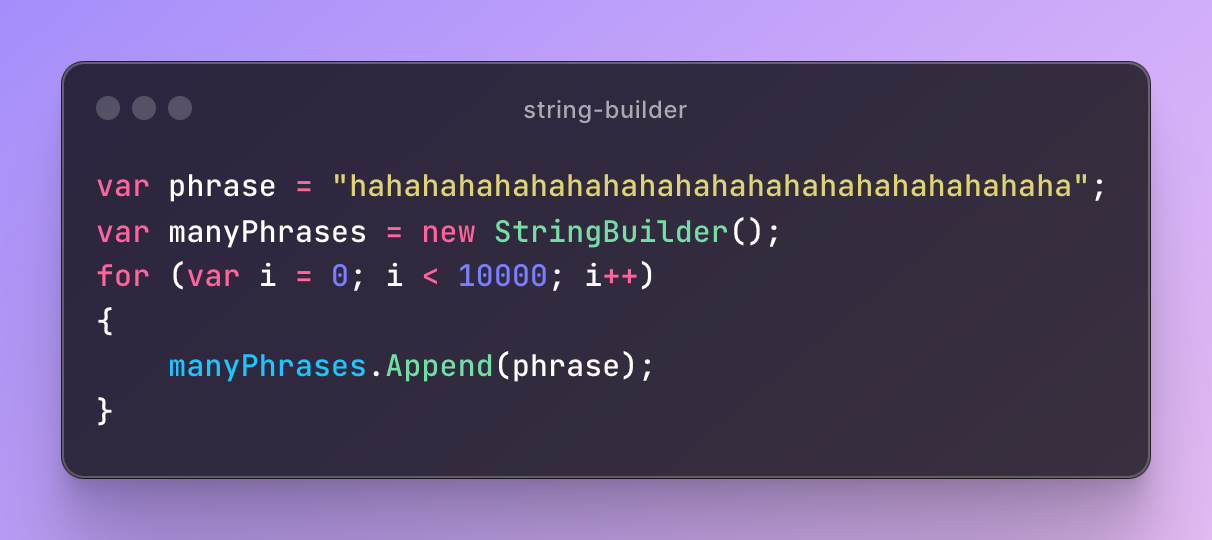
Also, Here are some naming conventions for identifier names that you should use throughout the .NET APIs.
- Use
PascalCase
for type names, namespaces, and all public members - Interface names should start with a capital
I
. - Use
camelCase
for method parameters and local variables. - Use meaningful and descriptive names for variables, methods, and classes.
- Use language keywords instead of BCL types (e.g.
int, string, float
instead ofInt32, String, Single
, etc) for both type references as well as method calls (e.g.int.Parse
instead ofInt32.Parse
).
These are some of the conventions that I found to be most important but you can still go through this .NET documentation to get a better idea about all the coding conventions that Microsoft suggests.