A string is an object of type String whose value is text. In C#, the string keyword is an alias for the String class.
C# provides many methods for creating, manipulating, and comparing strings. Let’s look at some of the most commonly used string methods below.
Clone()
This method helps to make a clone of a string that returns another copy of the same string.
string s1 = "CheeseBlogger";
// using Clone() method
string s2 = s1.Clone();
Console.WriteLine("Clone String : {0}", s2);
// Output-> "Clone String : CheeseBlogger"
Contains()
This method checks whether a specified character or string exists in the base string.
string s1 = "CheeseBlogger";
// using Contains() method
bool result = s1.Contains("m");
Console.WriteLine("Result : {0}", result);
// Output-> "Result : false"
Equals()
This method helps in comparing two strings. It returns true if both strings are the same.
string s1 = "CheeseBlogger";
// using Equals() method
string s2 = "Cheese Blogger";
bool result = s1.Equals(s2);
Console.WriteLine("Result : {0}", result);
// Output-> "Result : false"
Insert()
This method returns a new string in which a given string is inserted at a specified index position.
string s1 = "CheeseBlogger";
// using Insert() method
string s2 = s1.Insert(6, ' ');
Console.WriteLine(s2);
// Output-> "Cheese Blogger"
Split()
This method splits the string into a string array based on the specified value.
string s1 = "Cheese Blogger";
// using Split() method
string[] s2 = s1.Split(' ');
Console.WriteLine(s2);
// Output-> "["Singh", "Sharp"]"
ToCharArray()
This method converts a string into a char array.
string s1 = "CheeseBlogger";
// using ToCharArray() method
string[] s2 = s1.ToCharArray();
Console.WriteLine(s2);
// Output-> "["C", "h", "e", "e", "s", "e", "B", "l", "o", "g", "g", "e", "r"]
ToLower()
This method returns a copy of the string converted to lowercase.
string s1 = "CheeseBlogger";
// using ToLower() method
string s2 = s1.ToLower();
Console.WriteLine(s2);
// Output-> "cheeseblogger"
ToUpper()
This method returns a copy of the string converted to uppercase.
string s1 = "CheeseBlogger";
// using ToUpper() method
string s2 = s1.ToUpper();
Console.WriteLine(s2);
// Output-> "CHEESEBLOGGER"
Trim()
This method returns a new string in which all leading and trailing occurrences of a specified character from the current string are removed.
string s1 = "**CheeseBlogger**";
// using Trim() method
string s2 = s1.Trim('*');
Console.WriteLine(s2);
// Output-> "CheeseBlogger"
So here are some commonly used string methods we use in C#. Do you use any other method? Let me know in the comments below. Also, if you want to learn about contextual keywords in C# then read this blog.
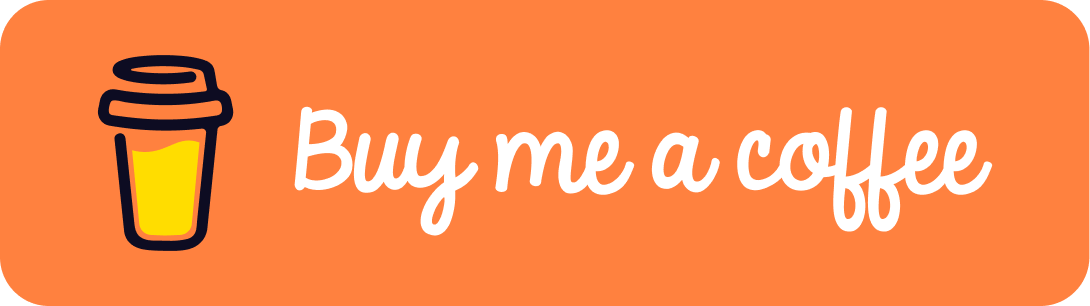
Pingback: Say Goodbye to multiple If Else CheeseBlogger.com